While the terms repetition and iteration are very descriptive words, the common term to describe these control structures is loop. Loops consist of two logical parts; the condition (i.e. The logic that evaluates the condition), and the loop body (i.e. Where the code integral to the loop is located). Assume your program contains a variable x, which is a valid positive integer. Write a for loop that prompts the user for x test scores. The prompts should indicate the number of the score being asked for, such as “Enter score #1: ”, “Enter score #2:”, etc.
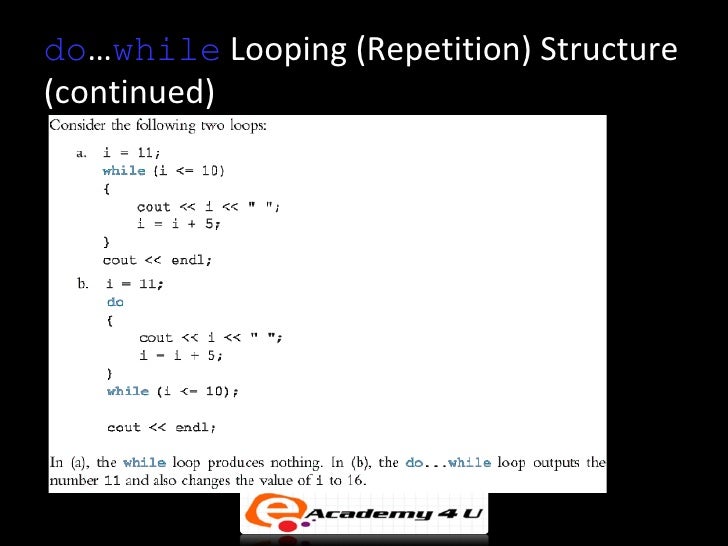
Which Of The Following Is A Repetition Structure In C++ Different
A(n) ____-controlled while loop uses a bool variable to control the loop. | Flag |
A do…while loop is a post-test loop. | true |
What is the output of the following C++ code? num = 10; | 10 |
The statement in the body of a while loop acts as a decision maker. | False |
The function eof returns true if the program has read past the end of the input file | True |
What is the next Fibonacci number in the following sequence? 1, 1, 2, 3, 5, 8, 13, 21, … | 34 |
The ____ statement can be used to eliminate the use of certain bool variables in a loop. | Break |
A break statement is legal in a while loop, but not in a for loop. | False |
The body of a do…while loop may not execute at all. | False |
The do…while loop has an exit condition but no entry condition. | True |
Consider the following code. int limit; cin >> limit; while (counter < limit) This code is an example of a(n) ____ while loop. | Counter Controlled |
In ____ structures, the computer repeats particular statements a certain number of times depending on some condition(s). | Looping |
It is possible that the body of a while loop may not execute at all, but the body of a for loop executes at least once. | False |
Which of the following loops is guaranteed to execute at least once? | do while loop |
What is the value of x after the following statements execute? | 40 |
Consider the following code. (Assume that all variables are properly declared.) cin >> ch; while (cin) This code is an example of a(n) ____ while loop. | … |
____ loops are called post-test loops. | do – while |
The control statements in the for loop include the initial statement, loop condition, and update statement. | True |
What is the output of the following C++ code? count = 1; | 25 1 |
A loop that continues to execute endlessly is called a(n) ____ loop. | Infinite |
In a sentinel-controlled while loop, the body of the loop continues to execute until the EOF symbol is read. | False |
In a sentinel-controlled while loop, the body of the loop continues to execute until the EOF symbol is read. | False |
*Which executes first in a do…while loop? | *The statement |
Loop control variables are automatically initialized in a loop. | False |
Both while and for loops are pre-test loops. | True |
When a continue statement is executed in a ____, the update statement always executes. | For loop |
In a counter-controlled while loop, the loop control variable must be initialized before the loop. | True |
Which of the following is a repetition structure in C++? | do while |
Suppose sum and num are int variables, and the input is 18 25 61 6 -1. What is the output of the following code? sum = 0; | 110 |
The number of iterations of a counter-controlled loop is known in advance. | True |
The execution of a break statement in a while loop terminates the loop. | True |
A sentinel-controlled while loop is an event-controlled while loop whose termination depends on a special value. | True |
The fourth number in a Fibonacci sequence is found by taking the sum of the previous two numbers in the sequence. | True |
After a break statement executes, the program continues to execute with the first statement after the structure. | True |
If a continue statement is placed in a do…while structure, the loop-continue test is evaluated immediately after the continue statement. | True |
A for loop is typically called an indexed for loop. | True |
Suppose sum, num, and j are int variables, and the input is 4 7 12 9 -1. What is the output of the following code? cin >> sum; | 2.24 1.25 |
Assume all variables are properly declared. What is the output of the following C++ code? num = 100; | 155 |
What is the output of the following loop? count = 5; | This is an infinite loop. |
Which of the following loops does not have an entry condition? | A Do While Loop |
The control variable in a flag-controlled while loop is a bool variable. | True |
What is the output of the following C++ code? int j; | 11 11 |
Suppose j, sum, and num are int variables, and the input is 26 34 61 4 -1. What is the output of the code? sum = 0; | 125 |
Which of the following is true about a do…while loop? | … |
Assume all variables are properly declared. The following for loop executes 20 times. for (i = 0; i <= 20; i++) | false |
An infinite loop may execute indefinitely. | True |
In the for statement, if the loop condition is omitted, it is assumed to be false. | False |
In the case of the sentinel-controlled while loop, the first item is read before the while loop is entered. | True |
A syntax error will result if the control statements of a for loop are omitted. | False |
An EOF-controlled while loop is another name for a sentinel-controlled while loop. | False |
Assume all variables are properly declared. The output of the following C++ code is 2 3 4 5. n = 1; | True |
What executes immediately after a continue statement in a while and do-while loop? | Loop Continue Test |
A loop is a control structure that allows you to repeat a set of statements until certain conditions are met. | True |
Which of the following is the initial statement in the following for loop? (Assume that all variables are properly declared.) int i; | … |
The following while loop terminates when j > 20. j = 0; | False |

Unfinished tasks keep piling up?
+Structure+(continued).jpg)
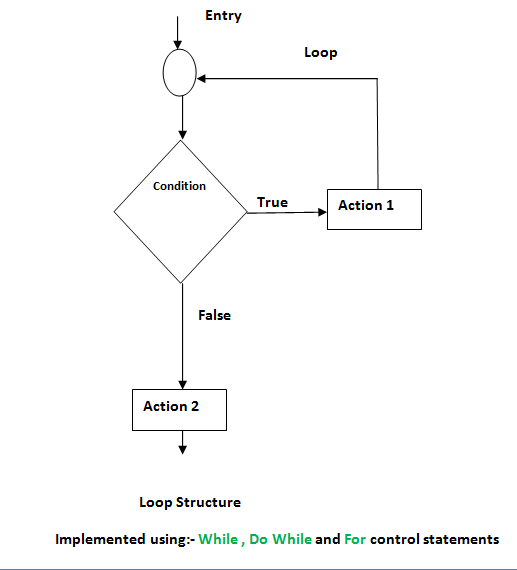
Let us complete them for you. Quickly and professionally.